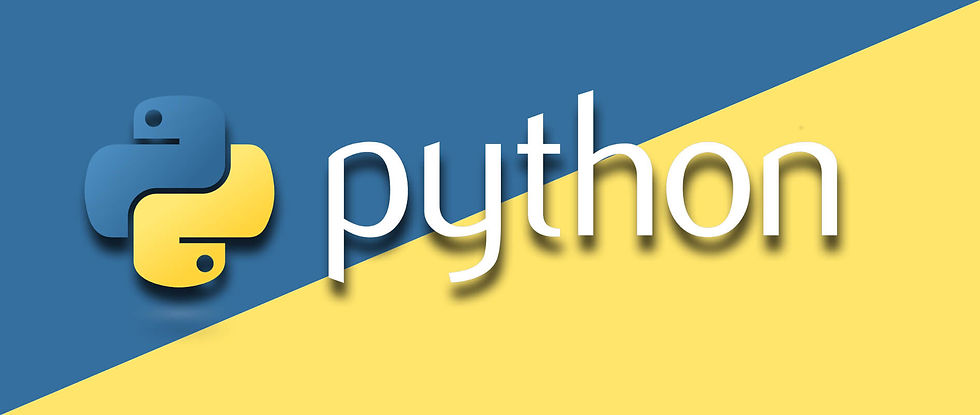
Computer shutdown prank
You may copy and paste it
# import the time module
import time
# define the countdown func.
def countdown(t):
while t:
mins, secs = divmod(t, 60)
timer = '{:02d}'.format(secs)
print(timer, end="\r")
time.sleep(1)
t -= 1
print('Shutting down...')
quit()
# function call
print('Computer Breakdown... Shutting down...')
countdown(int(10))
Live temperature(requires bs4)
You may copy and paste it
from bs4 import BeautifulSoup
import requests
import os
old_value =20
def clearconsole():
command = 'clear'
if os.name in ('nt', 'dos'): # If Machine is running on Windows, use cls
command = 'cls'
os.system(command)
while True:
soup = BeautifulSoup(requests.get(F"https://www.google.com/search?q=weather+singapore").content, 'html.parser')
list1 = soup.find_all('div')
x=list1[25].get_text()
temp = x[19:21]
if(int(temp) != int(old_value)):
old_value = int(temp)
clearconsole()
print('The temperature is ' + temp + '\u00b0C')
Guess the number
You may copy and paste it
import random
a = random.randint(0,100)
input1 = input('Please guess your number: ')
if int(input1) != a:
print('Sorry, you guessed incorrectly.')
print(f'Clue: It is from {a-random.randint(7,13)} to {a+random.randint(7,13)}')
input2 = input('Please guess your number: ')
if int(input2) != a:
print('Sorry, you guessed incorrectly.')
print(f'Clue: It is from {a-random.randint(3,6)} to {a+random.randint(3,6)}')
input3 = input('Please guess your number: ')
if int(input3) != a:
print('Sorry, you guessed incorrectly.')
print(f'Clue: It is from {a-random.randint(1,3)} to {a+random.randint(1,3)}')
input4 = input('Please guess your number: ')
if int(input4) != a:
print(f'The number is {a}.')
if int(input1) or int(input2) or int(input3) or int(input4) == a:
print('Congratulations!')
Security Checker
You may copy and paste it
while True:
try:
list1 = []
a = input('How many options each digit of code has: ')
b = input('How many digits of code do you have: ')
for i in range(int(a)*int(b)):
list1.append(str(i))
ab = (int(a)**int(b))**-1
z=str(ab)[-2::]
abc = ab*10**int(z)
q = abc/10
zq = str(q)[:2]
qz = str(q)[2::]
gibberish = zq+"0"*int(z)+qz
print(f'The chances of someone guessing your password is {gibberish}% or 1 in {int(a)**int(b)}.')
except:
print('There was an error')
Scissors, Paper, Stone
You may copy and paste it
import random
a = random.randint(1,3)
ab = input('Please type your option: ')
if a == 1:
b='🥌'
elif a ==2:
b ='📄'
else:
b ='✂️'
if ab == 'rock':
bab = '🥌'
elif ab == 'paper':
bab = '📄'
else:
bab = '✂️'
if ab == 'rock':
ba = 1
elif ab == 'paper':
ba = 2
else:
ba = 3
if ba==3 and a == 1:
print('Computer Won!')
print('Computer vs You')
print(f' {b} vs {bab}')
elif ba ==1 and a == 3:
print('You won!')
print('Computer vs You')
print(f' {b} vs {bab}')
elif ba<a:
print('Computer won!')
print('Computer vs You')
print(f' {b} vs {bab}')
elif a<ba:
print('You won!')
print('Computer vs You')
print(f' {b} vs {bab}')
else:
print('Tie!')
print('Computer vs You')
print(f' {b} vs {bab}')
Safe Password Generator
You may copy and paste it
import random
def repeat():
while True:
list = []
a = input('How many digits do you want your password to be: ')
for i in range(int(a)):
a = random.randint(1,26)
if a ==1:
list.append('a')
elif a ==2:
list.append('b')
elif a ==3:
list.append('c')
elif a ==4:
list.append('d')
elif a ==5:
list.append('e')
elif a ==6:
list.append('f')
elif a ==7:
list.append('g')
elif a ==8:
list.append('h')
elif a ==9:
list.append('i')
elif a ==10:
list.append('j')
elif a ==11:
list.append('k')
elif a ==12:
list.append('l')
elif a ==13:
list.append('m')
elif a ==14:
list.append('n')
elif a ==15:
list.append('o')
elif a ==16:
list.append('p')
elif a ==17:
list.append('q')
elif a ==18:
list.append('r')
elif a ==19:
list.append('s')
elif a ==20:
list.append('t')
elif a ==21:
list.append('u')
elif a ==22:
list.append('v')
elif a ==23:
list.append('w')
elif a ==24:
list.append('x')
elif a ==25:
list.append('y')
else:
list.append('z')
new_s = "".join(list)
print(new_s)
repeat()
String Switcher/Number Calculator
You may copy and paste it
initalinput = input('Please type 1(reverse) or 2(calculate): ')
if initalinput == 1:
a = input('Please type your number to reverse: ')
b = a[::-1]
print(b)
else:
c = input('Please type your order of operation: ')
d = input('Please type your 1st digit: ')
e = input('Please type your 2nd digit: ')
if c == '+':
print(int(d)+int(e))
elif c == '-':
print(int(d)-int(e))
elif c == '*':
print(int(d)*int(e))
elif c == '/':
print(int(d)/int(e))
elif c == '**':
print(int(d)**int(e))
Rolling Ball
You may copy and paste it
import os
import time
def clearconsole():
command = 'clear'
if os.name in ('nt', 'dos'): # If Machine is running on Windows, use cls
command = 'cls'
os.system(command)
for i in range (175):
print(f'💨{" "*i}⚽')
clearconsole()
Question Saver
You may copy and paste it
import os
list1=[]
def clearconsole():
command = 'clear'
if os.name in ('nt', 'dos'):
command = 'cls'
os.system(command)
clearconsole()
a = input('What is your question: ')
b = input('What is the answer to the question: ')
list1+=['Ans:' + b]
c = input('How many steps/explanations does the question have: ')
for i in range (int(c)):
if i == 0:
no = 'st'
elif i ==1:
no = 'nd'
elif i ==2:
no = 'rd'
else:
no='th'
d=input(f'What is the {i+1}{no} step/explanations: ')
list1+=[d]
clearconsole()
print(f'Qn:{a}')
Ans = input('Ans:')
if Ans != b:
print('Wrong!')
for i in range(len(list1)):
print(list1[i])
else:
print('Correct!')
Prime Number Finder
a=input('Enter a number(Enters the prime numbers between 1 to your number): ')
def isprime(e):
for i in range(2,e+1):
hello=True
for j in range(2,i):
abc=i%j
if abc==0:
hello=False
if hello==True:
print(i)
isprime(int(a))